Depth of Inheritance Tree (DIT)
The Depth of Inheritance Tree (DIT) metric measures the maximum inheritance path, referring to the number of levels a class is from the root class in an inheritance hierarchy. A deeper class hierarchy indicates a greater number of inherited methods and variables, which can contribute to increased complexity.
Inheritance is a tool to manage complexity, not to increase it. As a positive factor, deep trees promote reuse because of method inheritance.
A recommended DIT is 5 or less.
When a class inherits directly from Object
(or has no extends
), DIT=1.
Additional resources:
Config Example
To configure the depth-of-inheritance-tree
metric in DCM, specify the maximum DIT allowed for a class.
dart_code_metrics:
...
metrics:
...
depth-of-inheritance-tree: 5
...
In this configuration:
- The
depth-of-inheritance-tree
is set to 5, meaning any class with a DIT exceeding 5 will be flagged by different metric levels in DCM including[below, near, high, very high]
and if the value is 2x (or above) of the config, it will be marked asvery high
. - Adjust this value based on your project's specific requirements and complexity guidelines.
Why Be Cautious About DIT
There are several reasons to be careful about DIT, including:
- Complexity: A deep inheritance tree increases complexity and can make understanding the code more difficult.
- Maintenance: Deeper trees can make maintenance harder due to the larger number of inherited methods and variables.
- Fault-Proneness: Deep hierarchies can be more prone to faults as changes in base classes can have wide-reaching effects.
- Reuse vs. Overhead: While deep trees can promote reuse, they also increase the overhead of understanding and managing inherited properties and methods.
How Can You Improve?
- Limit Inheritance Levels: Try to keep the inheritance tree shallow to reduce complexity.
- Use Composition Over Inheritance: Prefer composition to inheritance where possible to avoid deep hierarchies.
- Refactor: Regularly refactor the class hierarchy to reduce unnecessary inheritance levels.
- Design Reviews: Conduct regular design reviews to maintain a clean and maintainable inheritance structure.
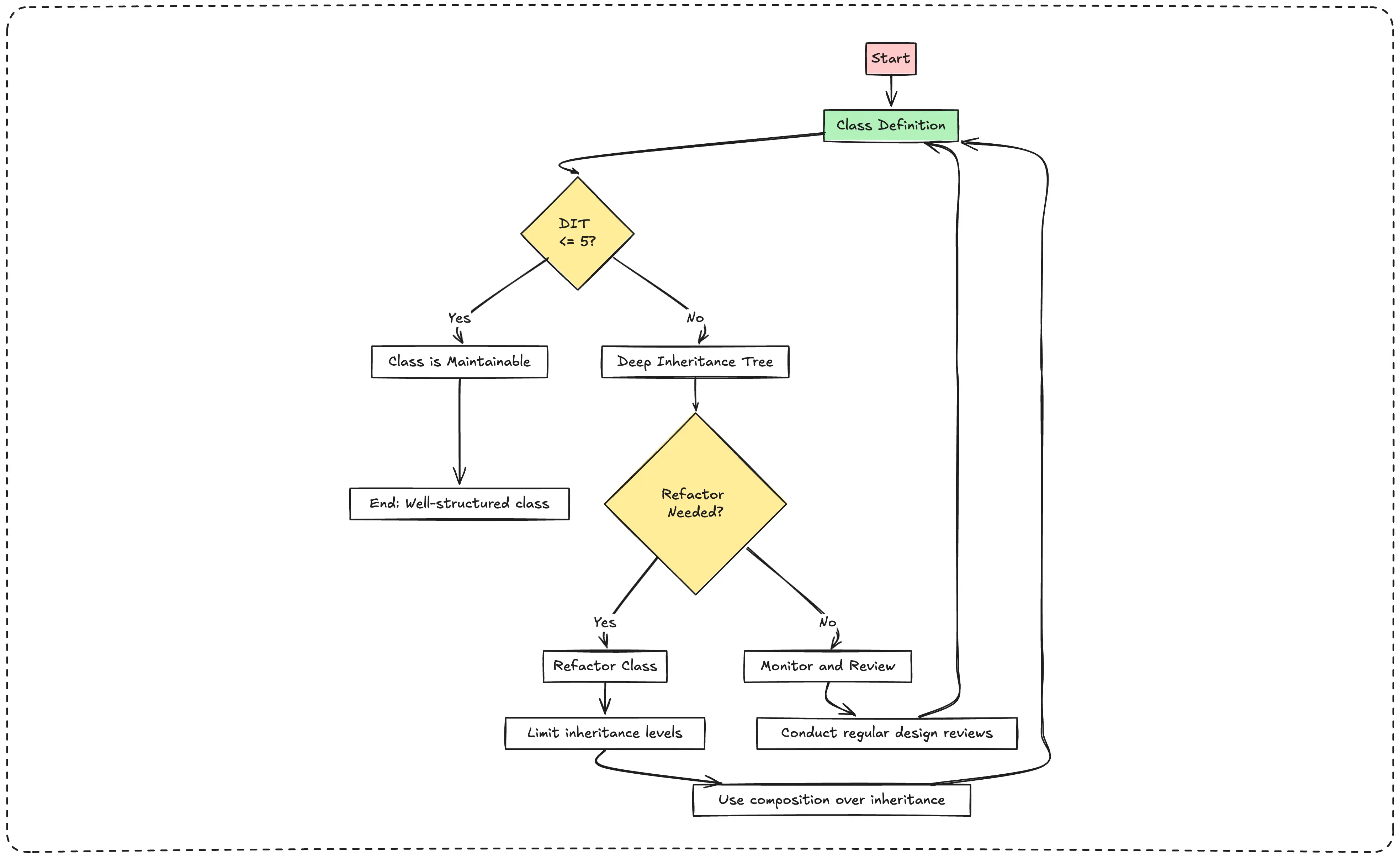
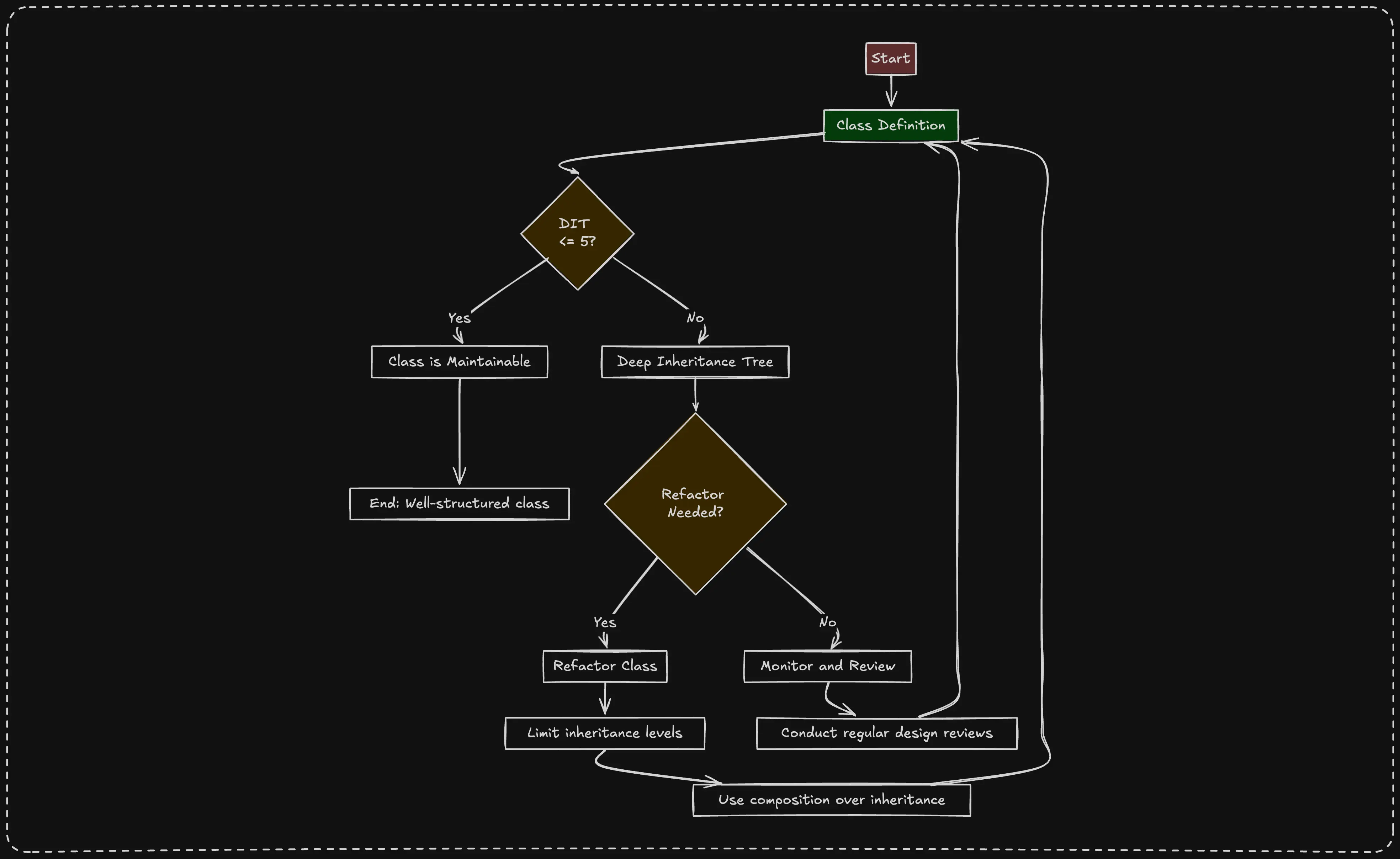
Example
❌ Bad: High Depth of Inheritance Tree (DIT)
class Animal {
void eat() {}
}
class Mammal extends Animal {
void breathe() {}
}
class Canine extends Mammal {
void bark() {}
}
class Dog extends Canine {
void fetch() {}
}
class ServiceDog extends Dog {
void assist() {}
}
// This class hierarchy has a DIT of 6, which is higher than the recommended DIT of 5.
class GuideDog extends ServiceDog {
void guide() {}
}
✅ Good: Low Depth of Inheritance Tree (DIT)
class Animal {
void eat() {}
}
class Mammal extends Animal {
void breathe() {}
}
class Dog extends Mammal {
void bark() {}
}
// This class hierarchy has a DIT of 4, adhering to the recommended DIT of 5 or less.
class ServiceDog extends Dog {
void assist() {}
}