Dart Code
Metrics
More than linter.
Configure in minutes, save hours.
Used by amazing teams
280+ configurable rules
dart_code_metrics:
rules:
- avoid-unused-parameters
- avoid-unrelated-type-assertions
- prefer-return-await
- always-remove-listener
- use-setstate-synchronously
- avoid-incomplete-copy-with
- dispose-fields
- proper-super-calls
- avoid-redundant-async
Quick fixes
- Quick Fix...
- Move first
- Ignore proper-super-calls for this line
- Ignore proper-super-calls for the entire file
- Fix all auto-fixable problems
- Extract...
- Extract Method
- Wrap With ...
Unused code and files detection
$: dcm check-unused-code lib
✔ Analysis is completed.
lib/main.dart:
⚠ unused function someFunction
at /path/to/file.dart:4:1
⚠ unused top level variable someVar
at /path/to/file.dart:6:1
⚠ unused class SomeClass
at /path/to/file.dart:8:1
22+ code metrics
dart_code_metrics:
metrics:
cyclomatic-complexity: 20
number-of-parameters: 4
maximum-nesting-level: 5
widgets-nesting-level: 5
number-of-used-widgets: 20
depth-of-inheritance-tree: 3
number-of-imports: 10
maintainability-index: 50
halstead-volume: 150
Used by amazing teams
Make fewer mistakes and speed up code reviews
DCM helps you spend less time on searching and fixing bugs, so you can focus more on what matters:
building great software.
1class _MyWidgetState extends State<MyWidget> {
2
3 Widget build(BuildContext context) {
4 setState(() {
5 myState = "Hello, Flutter!";
6 });
7
8 return Text(myState);
9 }
10}
Detect
problems
Find issues with 280+ lints
DCM statically analyzes your code with lint rules to quickly find problems and suggests fixes. The rules provided by DCM are not available in the built-in Dart SDK linter, but they are very handy.
Configure rules
All rules provided by DCM can be configured, so you can customize them to work exactly the way you need for your projects. You can also use rule presets to always have up to date rules list.
Apply
quick fixes
Via IDE code actions
Some rules provide a quick fix to help you address the issue faster. Applying several fixes at once via "fix all of type" or "fix all in a file" is also available.
From the console Teams
To speed up issues resolution even more, quick fixes can be applied to the entire project via "dcm fix".
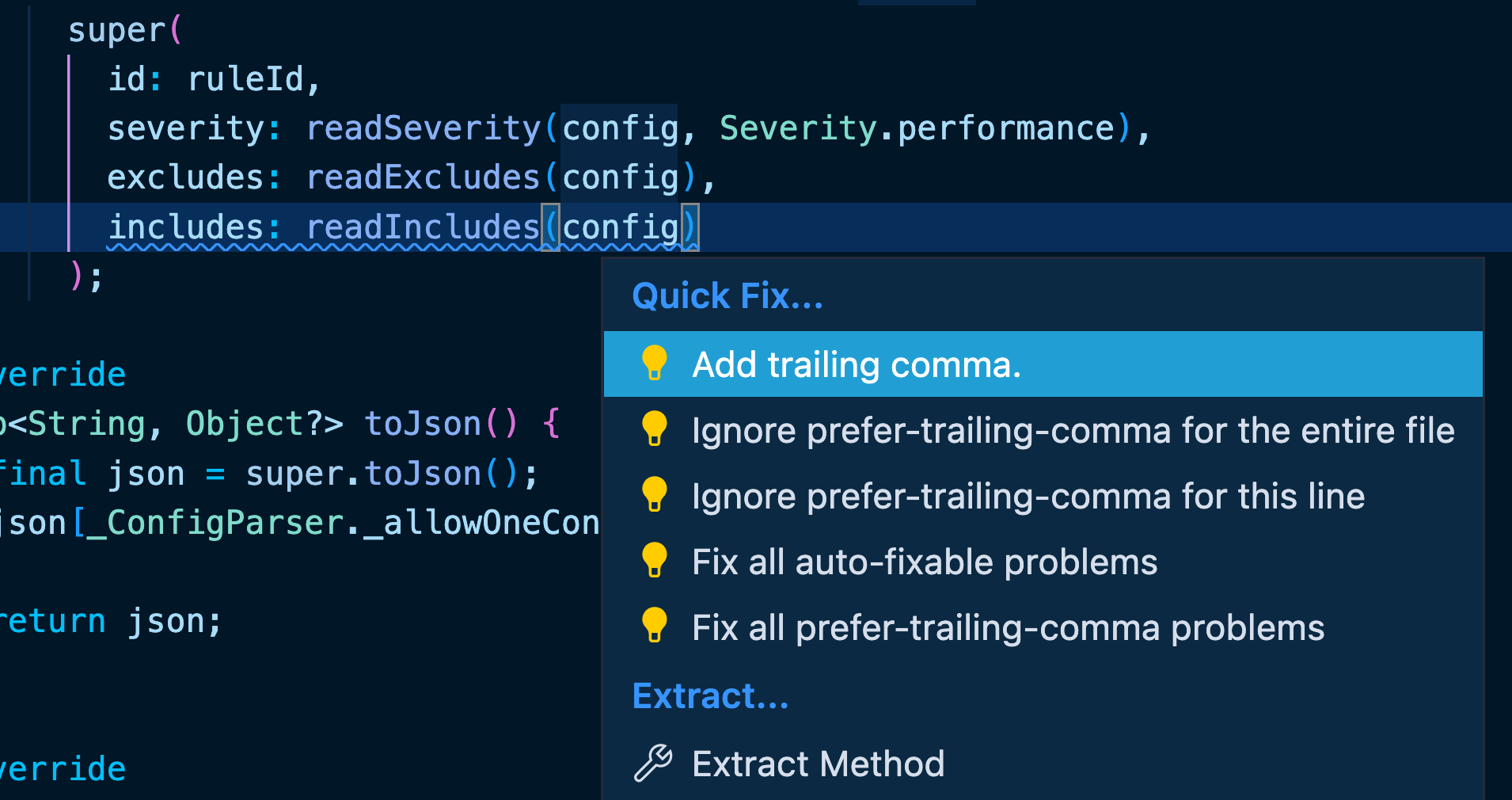
Detect
unused parts
Search unused code Teams
Even though the Dart compiler might optimize your code and exclude unused parts, maintaining such code is still your burden.
To find unused code in your codebase, try check-unused-code.
Search unused files
Want to be sure that all the files in your project are actually used? Try check-unused-files.
You can also automatically delete such files with the “--delete-files” option.
Search unused localization
Want to reduce your app bundle and amount of work for your localization team? Check if any localization in your codebase is unused.
Calculate
metrics
For existing projects Teams
Helps detect places in the codebase that need the most attention in terms of complexity / tech debt.
For new projects Teams
Provides instant feedback on pull requests, helping to ensure that code stays easy to maintain.
1dart_code_metrics:
2 metrics:
3 cyclomatic-complexity: 20
4 number-of-parameters: 4
5 maximum-nesting-level: 5
6 widgets-nesting-level: 5
7 number-of-used-widgets: 20
8 depth-of-inheritance-tree: 3
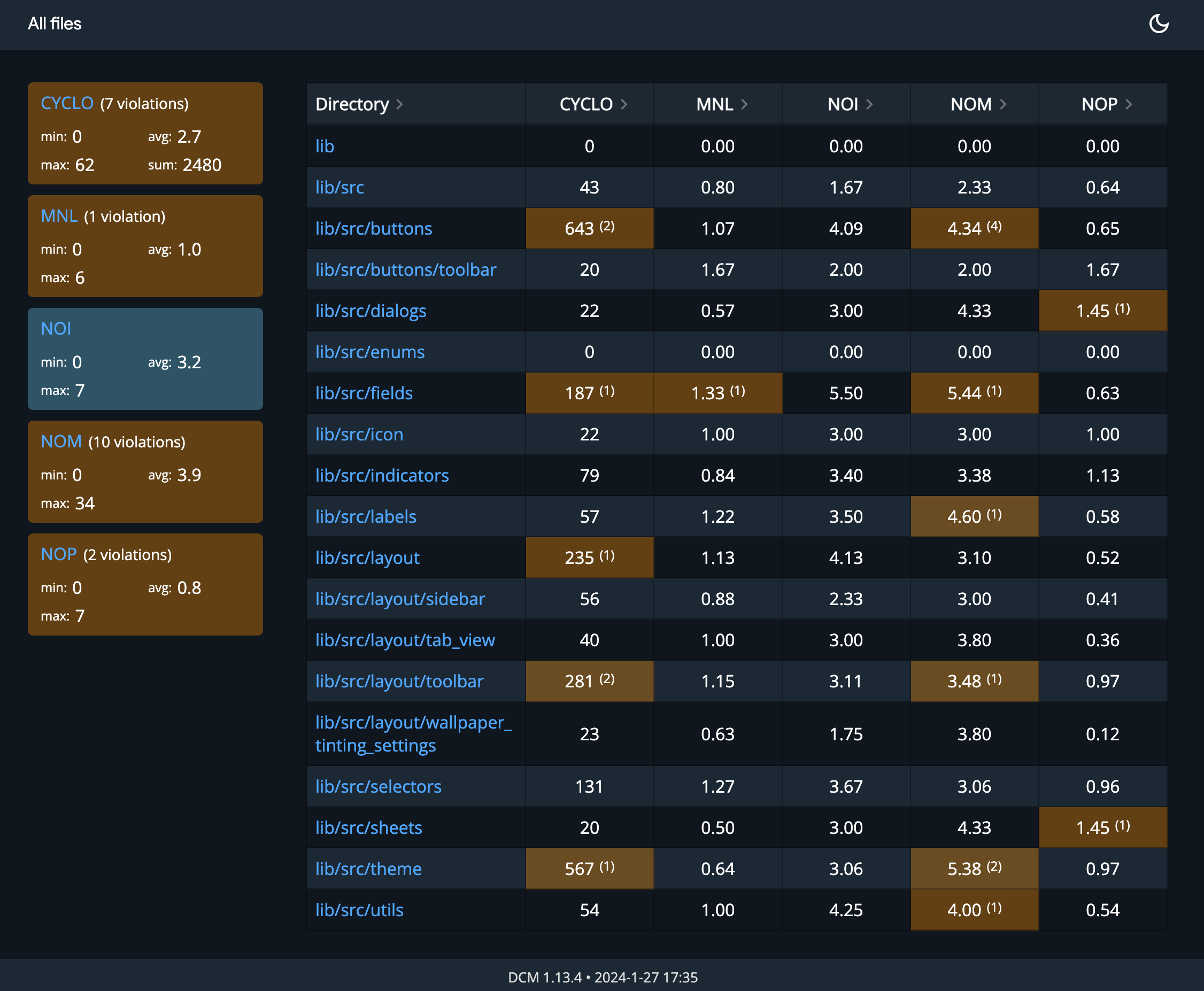
IDE integrations
Enable DCM for your favorite IDE.
What our users think
We're lucky to have a community that inspires us every day.
Read first-hand why people love DCM.
At Chili code quality is always a priority and adding DCM to our development flow elevated this process on several levels. Thanks to the abundant list of rules, a lot of potential problems are eliminated even before committing the code and getting to code review, which saves time and unnecessary back & forth. The DCM team is also very receptive to feedback and regularly releases new rules, as well as helps us with any issues. Our whole team is happy and unanimously agrees that DCM is a great productivity booster.
How DCM can help
your team?
For Developers
- Get fast feedback on your changes
- Avoid debugging tricky bugs
- Reduce the time spent on fixing bugs
- Work with standardized code in all projects
- Convince others to address tech debt
For Team Leads
- Speed up code reviews
- Gain visibility over codebase quality
- Improve reliability
- Improve release consistency
- Speed up onboarding for new developers
For Stakeholders
- Keep your users happy
- Rework less, innovate more
- Lower maintenance cost
- Reduce operability risk, avoid business disruption
- Improve release consistency
Ready to fix your code?
Start today